There are some really cool new
woodstock components available in the new VWP and from the java.net site. Woodstock is the original code name for the components which are used in VWP. These new components provide a new level of reusable web components which were not available before. One of the new components which is used extensively in project glassfish on the administrative console is the common tasks component.
This component provides a number of visual queues to what a user can do with the component. The component looks like this currently in Netbeans 6.0 M8. The component is not on the visual palette in 6.0 M9 Preview. The component does not necessarily look appealing in the current form on the palette, but renders perfectly.
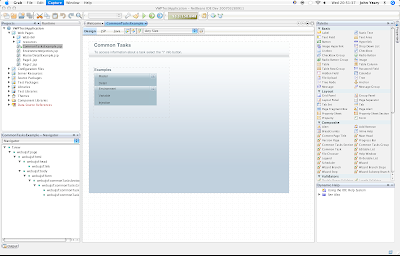
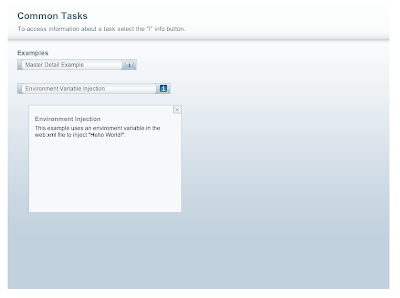
As you can see from the images this is a really cool idea. The info button "i" allows you to provide additional information about what the task does. You can add a URL for additional information and a separate title for the info that is displayed. Here is an example from
project glassfish where you can see another example of its use.
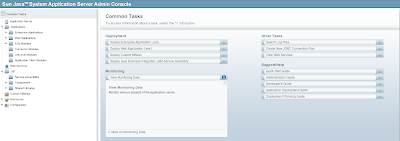
This shows you some of the capabilities.
The trick is how do you use it? On
project glassfish, when you click on the task it directs you to the appropriate category you selected. The details are scarce. So let me give you one solution. Use the actionExpression tag to set an action for the component. Here is mine for the example I showed above
<webuijsf:commontask
actionExpression="#{CommonTasksExample.masterDetailTaskAction}" binding="#{CommonTasksExample.commonTask1}"
id="commonTask1" infoLinkUrl="/faces/Page3.jsp"
infoText="This example shows how to use a DropDown component to set values in a table" infoTitle="Master-Detail Example"
style="height: 48px; width: 250px" target="_self" text="Master Detail Example"/>
The actionExpression is bound to a method which uses a navigation handler to navigate to the appropriate page.
public String masterDetailTaskAction() { getApplication().getNavigationHandler().handleNavigation(FacesContext.getCurrentInstance(), null, "MasterDetail");return null;}
This allows me to navigate as required. I hope this example will inspire you to try out the new components. They will add a level of professionalism to your web pages and make your users have a better experience on your site. Implemented fully, it may even avoid a call to the help desk, or you to figure out what a task is supposed to do.