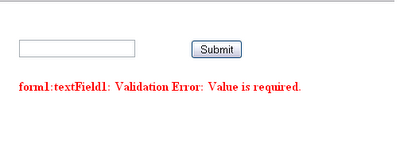
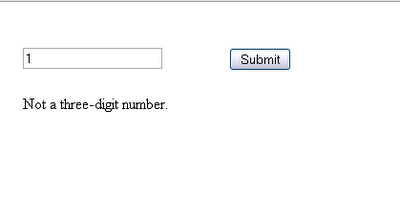
Here is a link to the Netbeans project: CustomValidatorExample.zip
1 /* 2 * 3 * Blue Lotus Software, LLC 4 * 5 * Copyright 2008. All Rights Reserved. 6 * 7 * $Id$ 8 * 9 */ 10 /* 11 * Copyright (C) 2008 Blue Lotus Software. All Rights Reserved. 12 * 13 * THIS SOFTWARE IS PROVIDED "AS IS," WITHOUT A WARRANTY OF ANY KIND. 14 * ALL EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, 15 * INCLUDING ANY IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A 16 * PARTICULAR PURPOSE OR NON-INFRINGEMENT, ARE HEREBY EXCLUDED. 17 * BLUE LOTUS SOFTWARE, LLC AND ITS LICENSORS SHALL NOT BE LIABLE 18 * FOR ANY DAMAGES OR LIABILITIES 19 * SUFFERED BY LICENSEE AS A RESULT OF OR RELATING TO USE, MODIFICATION 20 * OR DISTRIBUTION OF THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL 21 * BLUE LOTUS SOFTWARE, LLC OR ITS LICENSORS BE LIABLE FOR ANY LOST 22 * REVENUE, PROFIT OR DATA, OR FOR DIRECT, INDIRECT, SPECIAL, 23 * CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER CAUSED 24 * AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE 25 * OF OR INABILITY TO USE SOFTWARE, EVEN IF BLUE LOTUS SOFTWARE, LLC 26 * HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 27 * 28 * You acknowledge that Software is not designed, licensed or intended 29 * for use in the design, construction, operation or maintenance of any 30 * nuclear facility. 31 */ 32 package com.bluelotussoftware.j2se.examples; 33 34 import java.io.FileNotFoundException; 35 import java.io.IOException; 36 import java.io.RandomAccessFile; 37 import java.util.logging.Level; 38 import java.util.logging.Logger; 39 40 /** 41 * 42 * @author John Yeary 43 * @version 1.0 44 */ 45 public class RandomAccessFileExample { 46 47 private static final String CLASSNAME = RandomAccessFileExample.class.getName(); 48 private static Logger logger = Logger.getLogger(CLASSNAME); 49 50 /** 51 * @param args the command line arguments 52 */ 53 public static void main(String[] args) { 54 55 if (args.length < 1) { 56 System.err.print("You must provide at least a file name with path!"); 57 logger.log(Level.WARNING, "You must provide at least a file name with path"); 58 System.exit(0); 59 } 60 61 RandomAccessFile raf = null; 62 63 try { 64 raf = new RandomAccessFile(args[0], "rw"); 65 } catch (FileNotFoundException e) { 66 logger.log(Level.SEVERE, null, e); 67 // Error, set return to 1, and exit 68 System.exit(1); 69 } 70 71 for (int i = 1; i < args.length; i++) { 72 try { 73 raf.seek(raf.length()); 74 raf.writeBytes(args[i] + "\n"); 75 } catch (IOException e) { 76 logger.log(Level.SEVERE, null, e); 77 } 78 } 79 80 try { 81 raf.close(); 82 } catch (IOException e) { 83 logger.log(Level.SEVERE, null, e); 84 } 85 86 RandomAccessFileExample.readRAF(args[0]); 87 88 try { 89 raf = new RandomAccessFile(args[0], "rw"); 90 91 /* If you call seek() before you read, it will not work 92 * as expected. The read operation will move the cursor 93 * to the end of the read method. 94 */ 95 //raf.seek(0); 96 97 byte[] b = new byte[(int) raf.length()]; 98 raf.read(b); 99 100 /* Here we place the seek() method to append to 101 * start writing at the beginning of the file. 102 */ 103 raf.seek(0); 104 raf.writeBytes(CLASSNAME + "\n"); 105 106 // Append the original content of the file. 107 raf.write(b); 108 raf.close(); 109 } catch (IOException ex) { 110 logger.log(Level.SEVERE, null, ex); 111 } 112 RandomAccessFileExample.readRAF(args[0]); 113 } 114 115 public static void readRAF(String fileName) { 116 try { 117 RandomAccessFile raf = new RandomAccessFile(fileName, "r"); 118 String line = null; 119 while ((line = raf.readLine()) != null) { 120 System.out.println(line); 121 logger.log(Level.INFO, line); 122 } 123 } catch (FileNotFoundException ex) { 124 logger.log(Level.SEVERE, null, ex); 125 } catch (IOException ex) { 126 logger.log(Level.SEVERE, null, ex); 127 } 128 } 129 }
public static void deleteRAF(String value, String fileName) { try { RandomAccessFile raf = new RandomAccessFile(fileName, "rw"); long position = 0; String line = null; while ((line = raf.readLine()) != null) { System.out.println("line::line.length()::position::getFilePointer()"); System.out.println(line + "::" + line.length() + "::" + position + "::" + raf.getFilePointer()); logger.log(Level.INFO, line); if (line.equals(value)) { //Create a byte[] to contain the remainder of the file. byte[] remainingBytes = new byte[(int) (raf.length() - raf.getFilePointer())]; System.out.println("Remaining byte information::" + new String(remainingBytes)); raf.read(remainingBytes); //Truncate the file to the position of where we deleted the information. raf.getChannel().truncate(position); System.out.println("Moving to beginning of line..." + position); raf.seek(position); raf.write(remainingBytes); return; } position += raf.getFilePointer(); } } catch (FileNotFoundException ex) { logger.log(Level.SEVERE, null, ex); } catch (IOException ex) { logger.log(Level.SEVERE, null, ex); } }
java -classpath .:./lib/ExampleJar.jar TestCaseHere are the classes:
1 public class TestCase {
2
3 public TestCase() {
4 }
5
6 public static void main(String[] args) {
7 ClassA a = new ClassA();
8 a.print();
9 }
10 }
1 public class ClassA {
2
3 public void print() {
4 System.out.println("I am ClassA in a jar.");
5 }
6 }
1 public class ClassA {
2
3 // Bad apple!!!
4 public void print() {
5 System.out.println("I am ClassA in the wild...");
6 }
7 }
If I reverse the order to java -classpath lib/ExampleJar.jar:. TestCase
[sundev:Desktop:root]#java -classpath .:lib/ExampleJar.jar TestCase
I am ClassA in the wild...
[sundev:Desktop:root]#java -classpath lib/ExampleJar.jar:. TestCaseThe Classloader finds the first instance of the class which matches the appropriate signature and loads it. Subsequent classes of the same name and signature are not loaded.
I am ClassA in a jar.
java -cp "*"; TestCase (Windows)
java -cp '*' TestCase (Unix/OS X)
package com.bluelotussoftware.quartz.example;
/*
* HSQLJob.java
*
*/
/*
* Copyright (C) 2008 Blue Lotus Software. All Rights Reserved.
*
* THIS SOFTWARE IS PROVIDED "AS IS," WITHOUT A WARRANTY OF ANY KIND.
* ALL EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES,
* INCLUDING ANY IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A
* PARTICULAR PURPOSE OR NON-INFRINGEMENT, ARE HEREBY EXCLUDED.
* BLUE LOTUS SOFTWARE, LLC AND ITS LICENSORS SHALL NOT BE LIABLE
* FOR ANY DAMAGES OR LIABILITIES
* SUFFERED BY LICENSEE AS A RESULT OF OR RELATING TO USE, MODIFICATION
* OR DISTRIBUTION OF THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL
* BLUE LOTUS SOFTWARE, LLC OR ITS LICENSORS BE LIABLE FOR ANY LOST
* REVENUE, PROFIT OR DATA, OR FOR DIRECT, INDIRECT, SPECIAL,
* CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER CAUSED
* AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE
* OF OR INABILITY TO USE SOFTWARE, EVEN IF BLUE LOTUS SOFTWARE, LLC
* HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES.
*
* You acknowledge that Software is not designed, licensed or intended
* for use in the design, construction, operation or maintenance of any
* nuclear facility.
*/
/*
* Copyright 2008 John Yeary
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.sql.SQLException;
import java.sql.Statement;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import org.quartz.Job;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
/**
*
* @author John Yeary
* @version 1.0
*/
public class HSQLJob implements Job {
Connection con;
Statement s;
ResultSet rs;
ResultSetMetaData rsmd;
/** Creates a new instance of HSQLJob */
public HSQLJob() {
}
public void execute(JobExecutionContext context)
throws JobExecutionException {
try {
Class.forName("org.hsqldb.jdbcDriver");
} catch (Exception e) {
System.err.println("ERROR: failed to load HSQLDB JDBC driver.");
e.printStackTrace(System.err);
throw new JobExecutionException("Job Could not continue bacause driver failed to load");
}
System.out.println("Connecting to database...");
try {
// This may need to be changed to match the actual database name
con = DriverManager.getConnection("jdbc:hsqldb:hsql://localhost/glassfish", "sa", "");
} catch (SQLException e) {
throw new JobExecutionException("Failed to connect to HSQLDB Server");
}
try {
String sql = "SELECT * FROM INFORMATION_SCHEMA.SYSTEM_SESSIONINFO";
s = con.createStatement();
rs = s.executeQuery(sql);
rsmd = rs.getMetaData();
for (int i = 1; i <= rsmd.getColumnCount(); i++) {
System.out.print(rsmd.getColumnName(i) + "\t");
}
System.out.println();
while (rs.next()) {
for (int i = 1; i <= rsmd.getColumnCount(); i++) {
System.out.print(rs.getString(i) + "\t");
}
System.out.println();
}
// Clean up the connection
rs.close();
s.close();
con.close();
} catch (SQLException e) {
System.err.println(e.getMessage());
while (e.getNextException() != null) {
System.err.println(e.getMessage());
}
}
}
}
package com.bluelotussoftware.quartz.example;
/*
* QrtzScheduler.java
*
*/
/*
* Copyright (C) 2008 Blue Lotus Software. All Rights Reserved.
*
* THIS SOFTWARE IS PROVIDED "AS IS," WITHOUT A WARRANTY OF ANY KIND.
* ALL EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES,
* INCLUDING ANY IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A
* PARTICULAR PURPOSE OR NON-INFRINGEMENT, ARE HEREBY EXCLUDED.
* BLUE LOTUS SOFTWARE, LLC AND ITS LICENSORS SHALL NOT BE LIABLE
* FOR ANY DAMAGES OR LIABILITIES
* SUFFERED BY LICENSEE AS A RESULT OF OR RELATING TO USE, MODIFICATION
* OR DISTRIBUTION OF THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL
* BLUE LOTUS SOFTWARE, LLC OR ITS LICENSORS BE LIABLE FOR ANY LOST
* REVENUE, PROFIT OR DATA, OR FOR DIRECT, INDIRECT, SPECIAL,
* CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER CAUSED
* AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE
* OF OR INABILITY TO USE SOFTWARE, EVEN IF BLUE LOTUS SOFTWARE, LLC
* HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES.
*
* You acknowledge that Software is not designed, licensed or intended
* for use in the design, construction, operation or maintenance of any
* nuclear facility.
*/
/*
* Copyright 2008 John Yeary
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.text.ParseException;
import org.quartz.CronTrigger;
import org.quartz.JobDetail;
import org.quartz.Scheduler;
import org.quartz.SchedulerException;
import org.quartz.SchedulerFactory;
import org.quartz.impl.StdSchedulerFactory;
public class QrtzScheduler {
public static void main(String[] args) {
SchedulerFactory schedulerFactory = new StdSchedulerFactory();
Scheduler scheduler;
try {
scheduler = schedulerFactory.getScheduler();
scheduler.start();
JobDetail job = new JobDetail("hsqldb_example",
scheduler.DEFAULT_GROUP, HSQLJob.class);
CronTrigger cron = new CronTrigger("cron",
scheduler.DEFAULT_GROUP, "0/10 * * * * ?");
scheduler.scheduleJob(job, cron);
} catch (SchedulerException e) {
} catch (ParseException e) {
}
}
}
CREATE TABLE FAMILY (Now if I want to insert values from another table here is the query I used:
ID INT PRIMARY KEY GENERATED ALWAYS AS IDENTITY (START WITH 1, INCREMENT BY 1),
DESCRIPTION VARCHAR(50) NOT NULL
)
INSERT INTO FAMILY (DESCRIPTION) ( SELECT DISTINCT FAMILY FROM EQUIPMENT_GROUP WHERE FAMILY IS NOT NULL)Please note that I did not put anything into the ID column. If I try to specify a value, it will result in an exception
Since the column has been marked as GENERATED ALWAYS, it handles incrementing the key and assigning it.Exception: Attempt to modify an identity column 'ID'.
cp apache apache2
chgrp sys apache2Next change the flags on the file to execute
chmod u+x apache2Open the apache2 file with your favorite editor and modify the following lines:
APACHE_HOME=/usr/apache
CONF_FILE=/etc/apache/httpd.conf
RUNDIR=/var/run/apache
PIDFILE=${RUNDIR}/httpd.pid
APACHE_HOME=/usr/apache2
CONF_FILE=/etc/apache2/httpd.conf
RUNDIR=/var/run/apache2
PIDFILE=${RUNDIR}/httpd.pid
[dev1:init.d:root]#./apache2 startYou can check your configuration by using a browser to check it.
httpd starting.
ln -s ../init.d/apache2 K17apache2Go to /etc/rc1.d and add the following:
ln -s ../init.d/apache2 K17apache2Go to /etc/rc3.d and add the following:
ln -s ../init.d/apache2 S51apache2Go to /etc/rcS.d and add the following:
ln -s ../init.d/apache2 K17apache2
<jsp-config>
<!-- Set to true to disable JSP scriptiing syntax -->
<jsp-property-group>
<url-pattern>*.jsp</url-pattern>
<scripting-invalid>false</scripting-invalid>
</jsp-property-group>
<!-- Set to true to disable Expression Language (EL) syntax -->
<jsp-property-group>
<url-pattern>*.jsp</url-pattern>
<el-ignored>false</el-ignored>
</jsp-property-group>
</jsp-config>
1 <%@page contentType="text/html"%>
2 <%@page pageEncoding="UTF-8"%>
3 <%@ page import="java.util.Date" %>
4
5 <jsp:useBean id="now" scope="request" class="java.util.Date"/>
6
7 <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
8 "http://www.w3.org/TR/html4/loose.dtd">
9 <html>
10 <head>
11 <meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
12 <title>JSP Configuration</title>
13 </head>
14 <body>
15 <h1>JSP Configuration</h1>
16 <p>
17 Browser MIME types: ${header.accept}
18 </p>
19 <p>
20 Browser Compression: ${header["accept-encoding"]}
21 </p>
22 <p>
23 The context-path initParam is: ${initParam.customerServiceEmail}
24 </p>
25
26 <p>
27 HTTP Request Method: ${pageContext.request.method}<br>
28 HTTP Response Type: ${pageContext.response.contentType}<br>
29 HTTP Session ID: ${pageContext.session.id}<br>
30 HTTP Context Path: ${pageContext.servletContext.contextPath}
31 </p>
32 <p>
33 Date (script): <%= new Date()%><br>
34 Date(EL): ${now}
35 </p>
36 </body>
37 </html>
38
private String fx(String x) {
return x;
}
/**
*
* @param x
* @return
*/
private String fx(String x) {
return x;
}